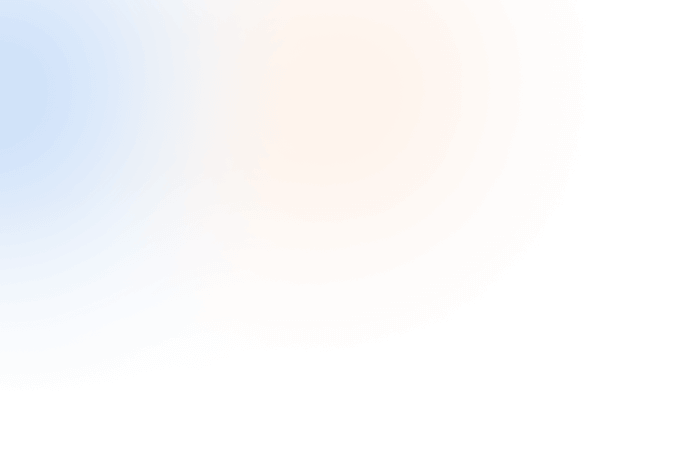
Entrepreneurial Thought Process and Decision-Making: A C++ Object-Oriented Programming Model
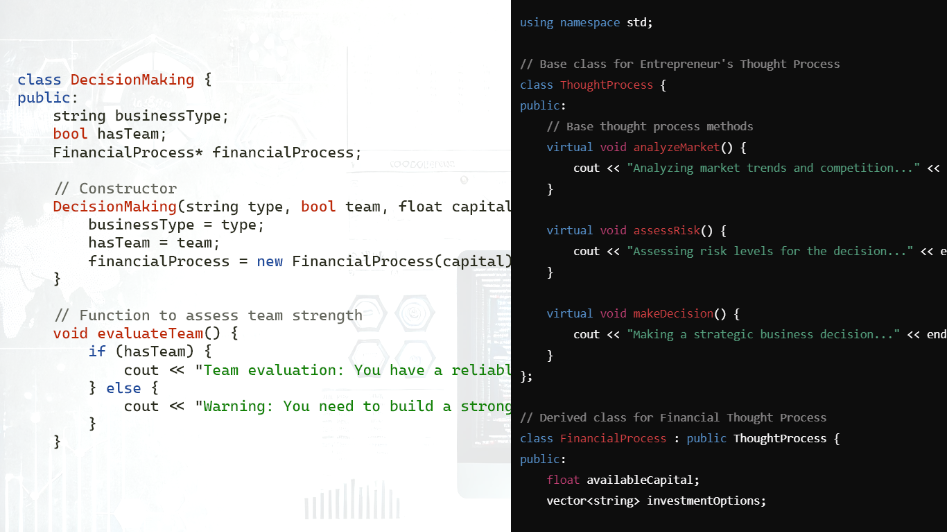
In the business world, entrepreneurs must navigate a complex decision-making process that includes market analysis, financial evaluation, risk assessment, and strategic planning. These processes resemble how a computer system would operate, making it ideal to model an entrepreneur's thought process using Object-Oriented Programming (OOP). By using classes, objects, loops, and decision-making structures like if-else, we can replicate the entrepreneurial mindset in C++.
Below is a breakdown of how the entrepreneurial decision-making process can be modeled through C++ code, leveraging OOP principles to structure the flow of thoughts, assessments, and outcomes.
Modeling Entrepreneurial Thought Process in C++ Using OOP
The following C++ code demonstrates how an entrepreneur makes decisions using financial and external factors. The process includes risk assessment, investment decisions, and team evaluation through the use of classes and functions, providing a structure to mimic human decision-making.
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// Base class for Entrepreneur's Thought Process
class ThoughtProcess {
public:
// Base thought process methods
virtual void analyzeMarket() {
cout << "Analyzing market trends and competition..." << endl;
}
virtual void assessRisk() {
cout << "Assessing risk levels for the decision..." << endl;
}
virtual void makeDecision() {
cout << "Making a strategic business decision..." << endl;
}
};
// Derived class for Financial Thought Process
class FinancialProcess : public ThoughtProcess {
public:
float availableCapital;
vector<string> investmentOptions;
// Constructor
FinancialProcess(float capital) {
availableCapital = capital;
investmentOptions = {"Tech Startup", "Real Estate", "Stock Market", "Cryptocurrency"};
}
void analyzeFinancials() {
cout << "Analyzing financial position, available capital: $" << availableCapital << endl;
}
void chooseInvestment() {
for (size_t i = 0; i < investmentOptions.size(); ++i) {
cout << "Option " << i + 1 << ": " << investmentOptions[i] << endl;
}
int choice;
cout << "Choose an investment option (1-4): ";
cin >> choice;
if (choice >= 1 && choice <= 4) {
cout << "You chose to invest in: " << investmentOptions[choice - 1] << endl;
} else {
cout << "Invalid option chosen, rethinking investment..." << endl;
}
}
// Overriding base class methods
void assessRisk() override {
cout << "Assessing financial risks for the investment decision..." << endl;
}
};
// Decision-Making Class that contains multiple processes (Primary Key)
class DecisionMaking {
public:
string businessType;
bool hasTeam;
FinancialProcess* financialProcess;
// Constructor
DecisionMaking(string type, bool team, float capital) {
businessType = type;
hasTeam = team;
financialProcess = new FinancialProcess(capital);
}
// Function to assess team strength
void evaluateTeam() {
if (hasTeam) {
cout << "Team evaluation: You have a reliable team to execute the strategy." << endl;
} else {
cout << "Warning: You need to build a strong team before proceeding." << endl;
}
}
// Function to execute the entrepreneurial decision process
void executeDecision() {
financialProcess->analyzeFinancials();
financialProcess->assessRisk();
financialProcess->chooseInvestment();
evaluateTeam();
// Final decision-making process
cout << "Final decision: Based on analysis, making the entrepreneurial decision..." << endl;
}
~DecisionMaking() {
delete financialProcess;
}
};
// External system or entity (Foreign Key)
class ExternalFactors {
public:
float economicCondition; // Economic condition rating out of 10
string regulatoryStatus; // Regulatory environment
ExternalFactors(float econCond, string regStatus) {
economicCondition = econCond;
regulatoryStatus = regStatus;
}
// Influence external factors on the decision-making process
void influenceDecision(DecisionMaking &dm) {
if (economicCondition < 5.0) {
cout << "Warning: Economic conditions are not favorable." << endl;
} else {
cout << "Economic conditions are stable." << endl;
}
if (regulatoryStatus == "Strict") {
cout << "Warning: Regulatory environment is strict. Adapt strategies accordingly." << endl;
} else {
cout << "Regulatory environment is business-friendly." << endl;
}
}
};
int main() {
// Entrepreneur's initial decision-making setup
string businessType = "Tech Startup";
bool hasTeam = true;
float availableCapital = 50000; // $50,000 in available capital
// Creating objects for Decision-Making process
DecisionMaking dm(businessType, hasTeam, availableCapital);
// External factors influencing decision
ExternalFactors externalFactors(6.5, "Business-Friendly");
// Process flow
cout << "Entrepreneur's Decision-Making Process Initiated..." << endl;
dm.executeDecision();
externalFactors.influenceDecision(dm);
return 0;
}
Key Concepts Explained:
Classes and Objects:
- ThoughtProcess, FinancialProcess, DecisionMaking, and ExternalFactors are modeled as classes to reflect different parts of the entrepreneur's thought process.
- Objects are created from these classes to execute the decision-making process based on specific data like available capital or market conditions.
Inheritance:
- FinancialProcess inherits from ThoughtProcess, adding specific financial decision-making logic to the basic thought process.
Control Flow with Loops:
- A for loop iterates over the investment options to help the entrepreneur decide on an investment.
If-Else Decision Structures:
- The evaluateTeam() and influenceDecision() functions use if-else logic to determine the entrepreneur's next steps based on team availability or external economic factors.
Primary and Foreign Keys:
- The DecisionMaking class serves as the primary process, handling the entrepreneur's core thought processes.
- ExternalFactors acts as the foreign key, representing external elements like economic conditions and regulations that influence the entrepreneur's decisions.
Bridging Entrepreneurship and Technology through Object-Oriented Programming
By using Object-Oriented Programming principles in C++, we can simulate the thought processes and decision-making flow of an entrepreneur. The entrepreneur’s decisions, much like a computer's, involve the analysis of data, assessment of risks, and application of logic to choose the best strategy for success. Using classes, functions, loops, and if-else conditions in programming mirrors the cognitive processes that go into effective business decision-making.
This model can be extended and scaled by adding more factors, such as competitive analysis, market trends, or detailed financial simulations, making it a valuable tool for understanding and implementing entrepreneurial strategies in a digital world.
For more insights on how startups are driving innovation and reshaping industries, check out our article on Startup Innovation: Fueling the Future of Business.
Start the conversation
Become a member of Bizinp to start commenting.
Already a member?
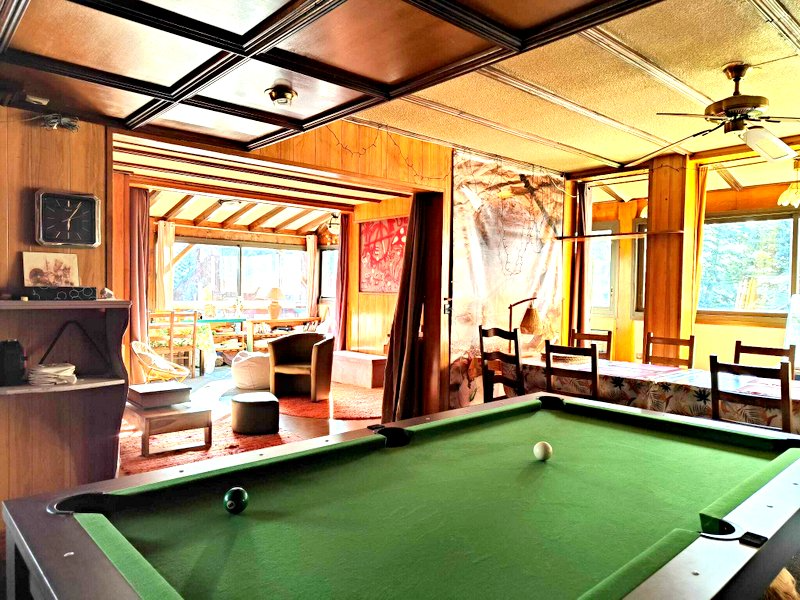
Small restaurant for sale in French Ski Resort
Great opportunity for people wishing to move to France on an entrepreneur visa. This small café/restaurant is located in the centre of one of France's most attractive ski resorts in the Pyrenees. It includes space for 40 diners, a fully-equipped professional kitchen and accommodation for the owner/manager. The very attractive price of €160,000 includes all the furniture and equipment as well as the agency fee: the business is ready to roll! More info directly from us at [email protected] There are two main seasons - Christmas through to end March (winter sports) and summer season from mid-June to the end of October (hiking, walking, nature clientele).